Drawing
You can click on the Open in workbench
button in most code samples to see (and
edit them) within the workbench.
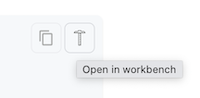
Let's start in two dimensions only, we will add the third one soon enough. replicad provides some classes and functions to draw in the plane.
Let's start with the powerful draw
API.
The draw
function and the Drawing API
With the drawing API you can draw straight lines and several types of curves. It currently supports:
- straight lines
- arcs of circles
- arcs of ellipses
- bezier curves
And for each of these categories it provides a set of functions that should help you draw stuff quickly - or give you as much power as you need. Have a look at the detailed API documentation to see what it can do
A simple drawing
Let's draw something simple:
const { draw } = replicad;
const main = () => {
return draw().hLine(25).halfEllipse(0, 40, 5).hLine(-25).close();
};
What have we done?
- We start drawing (at the origin, for instance
draw([10, 10])
would start at another point. - We then draw an horizontal line of 25 millimeters of length.
- Then, we draw an half ellipse, from the last point of the line, moving,
by
0
horizontally and by40
vertically - but drawing an arc of an ellipse with an axis length of5
. - We then go back of 25 horizontally
- We finally close the drawing, going from the current last point to the first point with a straight line.
Let's play with the drawing
To understand what the different parameters do, let's play with them:
- close with a mirror instead of a straight line with
.closeWithMirror
instead ofclose
- replace the second horizontal line by a sagitta line (an arc or circle)
.hSagittaArc(-25, 10)
- change the origin to another point (with
draw([10, 10])
for instance).
Drawing functions
In addition to the draw
API, replicad provides some drawing functions
to draw common and useful shapes. You can for instance:
- draw a rectangle
drawRoundedRectangle
- draw a polygon
drawPolysides
- circle
drawCircle
or ellipsedrawEllipse
- draw some text in a ttf font
drawText
- draw based on a parametric function
drawParametricFunction
, with an example here
They are documented in the API
Practicing with the watering can tutorial
You can have a look at a practical example of using the drawing API with the watering can tutorial